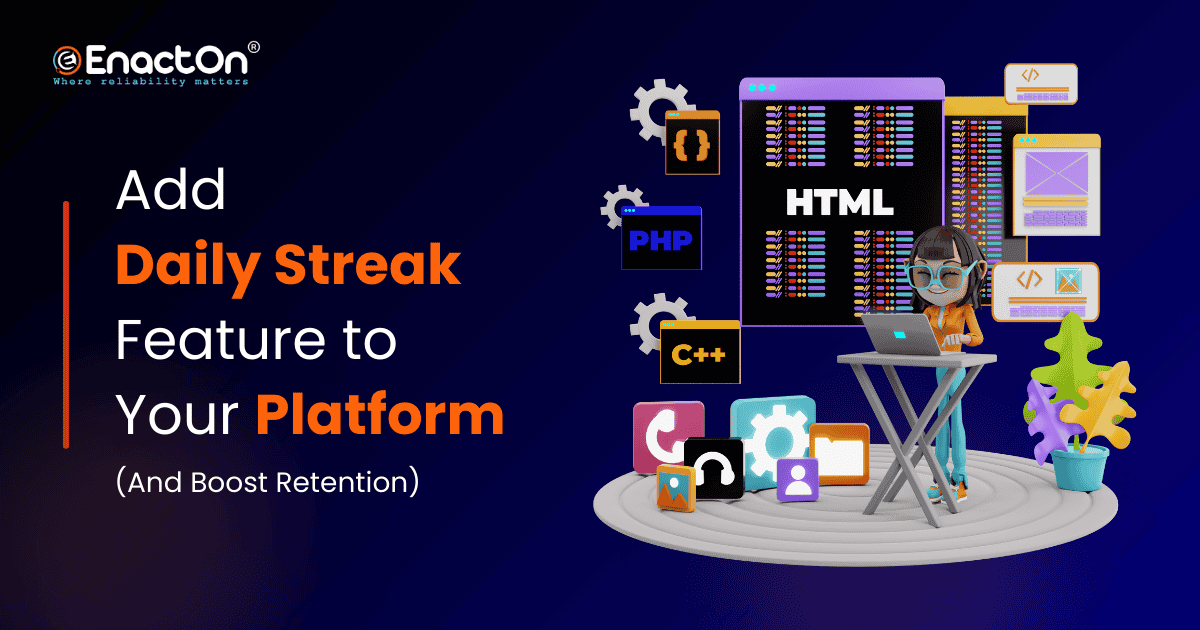
What keeps users hooked to an app, coming back day after day?
It’s not just great content or design; it’s the thrill of progress and rewards. Imagine opening an app daily, watching your streak grow, and earning bigger rewards as you hit new milestones.
It’s addictive, motivating, and incredibly powerful for boosting user engagement. In fact, this feature worked really well and helped Duolingo get over 17 million DAUs for their platform.
In this article, we’ll explore how you can build your very own daily rewards streak system—one that transforms casual users into loyal, daily visitors.
From understanding the psychology behind streaks and rewards to crafting a flexible, user-friendly experience, we’ll guide you through every step of development.
What is a Daily Streak?
A daily streak refers to a feature in applications that tracks how many consecutive days a user engages with the app. Every time a user completes a predefined action (such as logging in or completing a task) daily, their streak increases by one. However, if the user misses a day, the streak resets to zero.
Why Implement Daily Streak Feature?
Daily streaks are an effective way to boost user engagement. They encourage users to return to your platform consistently by offering rewards or incentives tied to maintaining the streak. Some key benefits of implementing the daily streak feature include:
- Increased User Retention: Users are more likely to return to your app to maintain their streak.
- Boosted User Motivation: The visual progress of a streak creates a sense of accomplishment.
- Gamification: Streaks add a fun, competitive element, especially when combined with rewards.
How Different Industries Can Use Daily Streak Rewards Feature?
- Fitness and Health Apps: Fitness apps could encourage users to log their workouts or meals daily. As a reward, users might get things like badges, discount coupons for fitness gear, or even a free month of premium features after hitting a certain number of consecutive days.
- Educational Platforms: To keep students motivated, educational platforms could reward them for completing daily lessons or quizzes. Rewards could be things like bonus content, points towards discounts on courses, or even digital certificates.
- Banking and Finance: Banks could get customers to use their apps daily by offering points for checking financial statuses or tracking daily spending. These points could then be used for perks like reduced service fees or better rates on savings accounts.
- E-Commerce: Online stores could encourage shoppers to log in every day by giving them access to exclusive flash sales or points that can be redeemed for discounts on future purchases.
- Streaming Services: These services could keep viewers coming back daily by rewarding them with access to exclusive content or periods of ad-free streaming after they log in several days in a row.
- Mobile and Video Games: Games can keep players engaged by offering daily rewards like in-game currency, special items, or early access to new content just for logging in.
- Online Publishing: Publishers could drive daily traffic by giving readers access to premium articles after a certain number of visits, or discounts on subscriptions
How to Create a Daily Streak Rewards for Your Platform?
Logic for daily streak rewards
- Start Streak: When a user first engages, start tracking the streak from “Day 1.”
- Daily Check: Every day, check if the user has logged in. If yes, increment the streak by 1. If they missed a day, reset the streak to “Day 1.”
- No Backtracking: Users cannot undo their progress; the streak can only move forward or reset.
- Complete and Reset: Once a user completes a streak (e.g., 7 or 10 days), they receive a reward, and the streak resets to “Day 1.”
- Maintain Consistency: The logic is designed to encourage daily activity, preventing users from skipping days or going back once the streak is started.
Step-by-Step Implementation for developing daily streak rewards feature
1. Setting up User Streak Data
The first step is to create a model or data structure to hold the streak-related information for a user. This will include:
- streakCount: The current streak the user has.
- lastActive: The last date the user interacted with the app.
- maxStreak: The maximum number of days needed to complete the streak.
Here’s an example of how this data might look:
const user = {
streakCount: 0, // Current streak count (number of consecutive active days)
lastActive: null, // Date of the user's last activity (e.g., '2024-10-13')
maxStreak: 7, // Maximum number of days for the streak (can be configured as needed)
};
Explanation:
- We initialize a user object that holds the necessary streak-related information.
- streakCount: Tracks how many consecutive days the user has maintained their streak. It is initialized to 0.
- lastActive: Stores the last date when the user engaged with the app. It is null initially, as the user hasn’t started the streak yet.
- maxStreak: The number of days the user must consecutively log in or perform an action to complete the streak. This is configurable and set to 7 days by default.
2. Time Management Logic
To track daily streaks, you need to compare the current date with the user’s last active date. The streak should only increment if the user logs in on the next consecutive day. If they miss a day, the streak resets.
Here’s how you could handle this:
function checkStreak(user) {
const currentDate = new Date(); // Get the current date
if (!user.lastActive) {
// First interaction, initialize the lastActive date and start the streak
user.lastActive = currentDate;
user.streakCount = 1;
} else {
const lastActiveDate = new Date(user.lastActive); // Convert lastActive to Date object
// Calculate the difference in days between current date and last active date
const differenceInDays = Math.floor((currentDate - lastActiveDate) / (1000 * 60 * 60 * 24));
if (differenceInDays === 1) {
// User logged in the next day, increment the streak
user.streakCount += 1;
} else if (differenceInDays > 1) {
// User missed one or more days, reset the streak
user.streakCount = 1;
}
}
// Update last active date to the current date
user.lastActive = currentDate;
}
Explanation:
- currentDate: Gets the current date when the function is called.
- The function checks if lastActive is null (i.e., it’s the first time the user is logging in):
- Sets lastActive to currentDate.
- Initializes streakCount to 1.
- If lastActive is already set:
- Calculates differenceInDays: Determines how many days have passed since the user’s last active date. The calculation converts the time difference from milliseconds to days.
- If differenceInDays equals 1: It means the user logged in the next day, so the streak is incremented by 1.
- If differenceInDays is greater than 1: The user missed one or more days, so the streak resets to 1.
- Finally, lastActive is updated to the current date.
3. Streak Progression and Reset
Now, we need logic to handle streak completion and reset. For example, if the streak reaches the maximum configured streak, we can reward the user and reset the streak.
function handleStreakProgress(user) {
// Check and update the user's streak
checkStreak(user);
// If the streak count reaches the maximum streak value
if (user.streakCount === user.maxStreak) {
console.log('Congrats! You completed your streak. Enjoy your reward!');
// Reset the streak after completion
user.streakCount = 0;
}
}
Explanation:
- handleStreakProgress(user): This function is called to manage the user’s streak.
- checkStreak(user): It first checks and updates the streak based on the user’s activity.
- If streakCount equals maxStreak, it means the user has completed their streak. A message is displayed congratulating the user, and the streak is reset to 0 to start over.
4. Making the Streak Configurable
If you want to make the streak duration configurable (for example, 7, 10, or 15 days), you can set this up dynamically:
function setMaxStreak(user, maxDays) {
// Set the maximum streak for the user
user.maxStreak = maxDays;
}
// Example usage: Set the maximum streak to 10 days
setMaxStreak(user, 10);
Explanation:
- setMaxStreak(user, maxDays): A utility function to set the maximum number of days required to complete a streak. This allows you to configure the streak duration easily.
- user.maxStreak = maxDays: Updates the user object with the desired maxDays value.
- setMaxStreak(user, 10): Sets the streak requirement to 10 days for this particular user.
5. Ensuring Users Can’t Claim Until Streak Completion
To prevent users from claiming rewards until the streak is completed, we can add a condition to check if the user’s streak is incomplete:
function canClaimReward(user) {
// Check if the user can claim the reward by comparing streakCount with maxStreak
return user.streakCount === user.maxStreak;
}
// Example usage
if (canClaimReward(user)) {
console.log('You can claim your reward!');
} else {
console.log(`You need to maintain your streak for ${user.maxStreak - user.streakCount} more days.`);
}
Explanation:
- canClaimReward(user): This function checks if the user has met the streak completion criteria.
- It returns true if the user’s streakCount matches maxStreak.
- Conditional Check:
- If canClaimReward(user) is true, a message indicates the user can claim their reward.
- If false, a message displays how many more days are needed to complete the streak.
6. Resetting the Streak
Once a user completes a streak, their streak resets to zero, and the process starts again from day 1.
function resetStreak(user) {
// Check if the user has completed the streak
if (user.streakCount === user.maxStreak) {
// Reset the streak count after completion
user.streakCount = 0;
console.log('Streak reset. Start from day 1 again!');
}
}
Explanation:
- resetStreak(user): A function to reset the user’s streak after completion.
- Checks if streakCount equals maxStreak.
- If true, the streak is reset to 0, and a message is displayed indicating that the streak has been reset.
Additional Considerations
- Tracking Daily Logins: You can choose to track user activity through logins, specific actions like completing tasks, or even page visits.
- Handling Time Zones: Make sure to handle time zone differences if you have a global user base. Use the user’s local time zone to calculate streaks correctly.
- User Notifications: You can notify users about streak status using in-app messages or emails. Notify them when they’re close to completing the streak or when they’ve missed a day.
Also read: How to Develop Daily Bonus Ladder Feature
Conclusion
Implementing a daily streak feature can have a tremendous impact on user engagement and retention in your application. By using simple JavaScript logic, you can configure streak lengths, handle streak progression, and reward users for maintaining their streaks.
With the flexibility to adjust streak durations and define rewards for streak completions, this feature offers a powerful gamification element to keep users motivated and engaged with your application.
Feel free to modify the code snippets provided to suit your platform’s architecture.